Description:-
In this Example we Explain that How to Create or Generate thumbnails from Images in Asp.Net.
First we Understand that what is the Actual meaning of thumbnails image are as follow:
What is Thumbnails:-
THUMBNAIL is a "Small version of a picture" Thumbnail is a one kind of term used by graphic designers and photographers for a small images to representation of a larger image.
The intention behind this is that to manage group of larger images so that people can easily look that images.
In this Examle we Create a one List and that list fetch Images one by one from image folder and store it. After Fetching all images we will assign this list to the DataList control so we can Easily Display Image in proper Layout.
Before Assign List Images to DataList Control we also convert this Image to an Thumbnails Format and store it and assign to DataList control.
to show Example of How to Bind Image in DataList with CRUD operation click here CRUD operation in DataList
to show Example of How to Create a Paging in DataList click here Paging in DataList
To DownLoad this Complete Project Click the Below Download Image
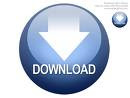
Thumbnailimage.aspx:-
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Thumbnailimage.aspx.cs" Inherits="Thumbnailimage" %>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Generate a Thumbnails of the Images in Asp.Net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:FileUpload ID="fileupload1" runat="server" /><br />
<asp:Button ID="btnadd" runat="server" Text="Upload Image" onclick="btnadd_Click" />
</div>
<div>
<b>List of the thumanails of the Images From
Image Folder</b>
</div>
<div>
<asp:DataList ID="datalist" runat="server" RepeatColumns="3" CellPadding="4" ForeColor="#333333">
<ItemTemplate>
<asp:Image ID="Image1" ImageUrl='<%# Bind("Name", "~/Images/{0}") %>' runat="server" />
<br />
<asp:HyperLink ID="HyperLink1" Text='<%# Bind("Name") %>' NavigateUrl='<%# Bind("Name", "~/Images/{0}") %>' runat="server"/>
</ItemTemplate>
<AlternatingItemStyle BackColor="White" />
<FooterStyle BackColor="#507CD1" Font-Bold="True" ForeColor="White" />
<HeaderStyle BackColor="#507CD1" Font-Bold="True" ForeColor="White" />
<ItemStyle BorderColor="Brown" BorderStyle="dotted" BorderWidth="3px" HorizontalAlign="Center"
VerticalAlign="Bottom" BackColor="#EFF3FB" />
<SelectedItemStyle BackColor="#D1DDF1" Font-Bold="True" ForeColor="#333333" />
</asp:DataList>
</div>
</form>
</body>
</html>
Thumbnailimage.aspx.cs:-
using System.Collections;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.IO;
using System;
public partial class Thumbnailimage : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindDataList();
}
}
protected void BindDataList()
{
DirectoryInfo dir = new DirectoryInfo(MapPath("Images")); //Images is the folder in
which all images are Uploaded
FileInfo[] files = dir.GetFiles();
ArrayList listItems = new ArrayList();
foreach (FileInfo info in files)
{
if (!info.ToString().Equals("Thumbs.db"))
listItems.Add(info);
}
datalist.DataSource = listItems;
datalist.DataBind();
}
protected void btnadd_Click(object sender, EventArgs e)
{
string filename = Path.GetFileName(fileupload1.PostedFile.FileName);
string targetPath = Server.MapPath("Images/" +
filename); // Uploaded Images in the images folder
Stream strm =
fileupload1.PostedFile.InputStream;
var targetFile = targetPath;
//Based
on scalefactor image size will vary
GenerateThumbnails(0.07, strm,
targetFile);
BindDataList();
}
private void GenerateThumbnails(double scaleFactor, Stream sourcePath, string targetPath)
{
using (var image = Image.FromStream(sourcePath))
{
var newWidth = (int)(image.Width * scaleFactor);
var newHeight = (int)(image.Height * scaleFactor);
var thumbnailImg = new Bitmap(newWidth, newHeight);
var thumbGraph = Graphics.FromImage(thumbnailImg);
thumbGraph.CompositingQuality = CompositingQuality.HighQuality;
thumbGraph.SmoothingMode = SmoothingMode.HighQuality;
thumbGraph.InterpolationMode = InterpolationMode.HighQualityBicubic;
var imageRectangle = new Rectangle(0, 0, newWidth, newHeight);
thumbGraph.DrawImage(image, imageRectangle);
thumbnailImg.Save(targetPath,
image.RawFormat);
}
}
}
0 comments:
Post a Comment