Description:-
In this
Example I Explain that How Create AutoComplete TextBox in Asp.Net Using
Webservice and Jquery.
Before we discuss any thing we first understand what
is webservice in asp.net
What is WebService:-
Web Service is one kind of
application that is designed to interact directly with other applications over
the internet. In simple sense, Web Services are means for interacting with another
objects that are already defined over the Internet.
in this example we provide Facility to user that is when user type any character in TextBox then It's Related Name are Automatically Bind it and Display to the user so user can easily Find the Record when the size of Record are in Lakhs.
this is the same Functionality as a google search engine we all know that when we type any character or word in Google then it will Automatically Display 10 same Result in Dropdown box so user can easily find it and also saving the time.
in this example we provide Facility to user that is when user type any character in TextBox then It's Related Name are Automatically Bind it and Display to the user so user can easily Find the Record when the size of Record are in Lakhs.
this is the same Functionality as a google search engine we all know that when we type any character or word in Google then it will Automatically Display 10 same Result in Dropdown box so user can easily find it and also saving the time.
Before using this Example first
Create a table in SqlServer Database with Following Field and name of table is
poll.
To Download this Example Click the Below Download Link
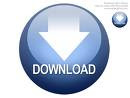
AutocompleteTextbox.aspx:-
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="AutocompleteTextbox.aspx.cs" Inherits="AutocompleteTextbox" %>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>AutoComplete
Textbox with webservice using jQuery</title>
<link href="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8.1/themes/base/jquery-ui.css" rel="stylesheet" type="text/css"/>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8.1/jquery-ui.min.js"></script>
<script type="text/javascript">
$(document).ready(function ()
{
SearchText();
});
function
SearchText() {
$(".autosuggest").autocomplete({
source: function
(request, response) {
$.ajax({
type: "POST",
contentType: "application/json;
charset=utf-8",
url: "AutoCompleteService.asmx/GetAutoCompleteData",
data: "{'username':'" +
document.getElementById('txtSearch').value
+ "'}",
dataType: "json",
success: function
(data) {
response(data.d);
},
error: function
(result) {
alert("Error");
}
});
}
});
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div class="demo">
<div class="ui-widget">
<label for="tbAuto">Enter
UserName: </label>
<input type="text" id="txtSearch" class="autosuggest" />
</div>
</form>
</body>
</html>
Look at this the above code that I defined in header section I
added some of javascript and Jquery and css files. by using those files we have
a chance to display auto complete text with css style and I mentioned url field
as “AutoCompleteService.asmx/GetAutoCompleteData”
this mean we are calling GetAutoCompleteData
method from AutoCompleteService.asmx
webservice.
To
create a webservice right click on your application -> Select Add New
Item -> select Web Service -> Give name of service AutoCompleteService.asmx
and click OK
now webservice File is created open code behind file
and write the following code that are Defined Below.
AutocompleteService.cs:-
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Web.Services;
using
System.Data.SqlClient;
using
System.Data;
/// <summary>
///
Summary description for AutoCompleteService
/// </summary>
[WebService(Namespace
= "http://tempuri.org/")]
[WebServiceBinding(ConformsTo
= WsiProfiles.BasicProfile1_1)]
// To allow this Web Service
to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class AutoCompleteService :
System.Web.Services.WebService {
public
AutoCompleteService () {
//Uncomment the following
line if using designed components
//InitializeComponent();
}
[WebMethod]
public string
HelloWorld() {
return "Hello
World";
}
[WebMethod]
public List<string>
GetAutoCompleteData(string username)
{
List<string>
result = new List<string>();
using (SqlConnection
con = new SqlConnection("Data
Source=SQLDB;Persist Security Info=True;User ID=Demoh;Password=Demo1@"))
{
using (SqlCommand
cmd = new SqlCommand("select
DISTINCT Name from poll where Name LIKE '%'+@SearchText+'%'",
con))
{
con.Open();
cmd.Parameters.AddWithValue("@SearchText",
username);
SqlDataReader dr
= cmd.ExecuteReader();
while
(dr.Read())
{
result.Add(dr["Name"].ToString());
}
return
result;
}
}
}
}
Check this....C# Autocpmplete textbox
ReplyDeleteLing
hi, is it possible to display the results as a clickable link?
ReplyDeletethanks
nice one. helped me
ReplyDelete