Description:-
In previous
Example I already Explain that how to send sms to any user from our asp.net
Application using way2sms or Fullonsms services. But now this service is not
working because ithis service is now not available so behind this result I found
the other services to send sms to any user from our Application. so to send the
sms first you have to perform the following the steps are follows
Using This service allowed multiple site like
Way2sms, FullOnSMS, 160by2, Ultoo.com, SmsSpark.com SMSFI.com Freesms8, Site2SMS,
IndyaRocks.com SMSAbc.com , Sms440, BhokaliSMS, etc.
In order to send sms you have to follow this
three steps
1. Register at Site2SMS and get Username and
Password.
2. Register at Mashape.com and generate key.
3. Download the .DLL file from ASPSnippets.SmsAPI.dll click here and add it in your project bin folder
Example of auto complete Textbox search Autocomplete Search in Ajax
we already add .dll file in a project to download this
to download complete example click here
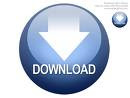
CS.aspx:-
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="CS.aspx.cs" Inherits="CS" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0
Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Send free SMS in ASP.Net using C# and
VB.Net</title>
<style type="text/css">
body {
font-family: Arial;
font-size: 10pt;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<table border="0">
<tr>
<td>Your Mobile Number:
</td>
<td>
<asp:TextBox ID="txtyourmoNumber" runat="server"></asp:TextBox>
</td>
<td>
<asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server" ErrorMessage="Required"
ControlToValidate="txtyourmoNumber" ForeColor="Red"></asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td>your site2sms Password:
</td>
<td>
<asp:TextBox ID="txtyourPassword" TextMode="Password" runat="server"></asp:TextBox>
</td>
<td>
<asp:RequiredFieldValidator ID="RequiredFieldValidator2" runat="server" ErrorMessage="Required"
ControlToValidate="txtyourPassword" ForeColor="Red"></asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td>Reciever mobile Number:
</td>
<td>
<asp:TextBox ID="txtRecieveNumber" runat="server" Width="300"></asp:TextBox>
</td>
<td>
<asp:RequiredFieldValidator ID="RequiredFieldValidator3" runat="server" ErrorMessage="Required"
ControlToValidate="txtRecieveNumber" ForeColor="Red"></asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td>Message Boby Text:
</td>
<td>
<asp:TextBox ID="txtMessage" runat="server" TextMode="MultiLine"></asp:TextBox>
</td>
<td>
<asp:RequiredFieldValidator ID="RequiredFieldValidator5" runat="server" ErrorMessage="Required"
ControlToValidate="txtMessage" ForeColor="Red"></asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td></td>
<td>
<asp:Button ID="btnSendsms" runat="server" Text="Send" OnClick="btnSend_Click" />
</td>
<td></td>
</tr>
</table>
</form>
</body>
</html>
CS.aspx.cs:-
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Web.UI;
using
System.Web.UI.WebControls;
using
System.Text;
using
System.Net;
using
System.IO;
using
ASPSnippets.SmsAPI;
public partial class CS : System.Web.UI.Page
{
protected void btnSend_Click(object sender, EventArgs e)
{
SMS.APIType = SMSGateway.Site2SMS;
SMS.MashapeKey = "<Mashape API
Key>";
SMS.Username = txtyourmoNumber.Text.Trim();
SMS.Password = txtyourPassword.Text.Trim();
if (txtRecieveNumber.Text.Trim().IndexOf(",") == -1)
{
//Single SMS
SMS.SendSms(txtRecieveNumber.Text.Trim(), txtMessage.Text.Trim());
}
else
{
//Multiple SMS
List<string> numbers =
txtRecieveNumber.Text.Trim().Split(',').ToList();
SMS.SendSms(numbers, txtMessage.Text.Trim());
}
}
}
thank you.......
ReplyDeletei get this error
ReplyDeleteThe underlying connection was closed: The connection was closed unexpectedly.
First Follow the step properly and create your account in Site2sms and check your Internet connection. it is working properly.
ReplyDeletehello sir
Deletei got error {"message":"Invalid Mashape Key"}
what can i do ??
please help me as soon as possible
Hi thank you for the article. I had a couple of easy questions. So if I follow those steps, will this be able to send out like 50 sms text in bulk pretty easily and maybe up to 1000 per day? Is this sms service free? Thank you in advance.
ReplyDeletethank you for comments............
Deleteno error but sms not sent
ReplyDeleteit's work properly plz follow this step properly and the try again
DeleteSend Text or bulk SMS in ASP.Net using C# and VB.Net
Posted on 02:30 by kirit kapupara with 7 comments
Description:-
In previous Example I already Explain that how to send sms to any user from our asp.net Application using way2sms or Fullonsms services. But now this service is not working because ithis service is now not available so behind this result I found the other services to send sms to any user from our Application. so to send the sms first you have to perform the following the steps are follows
Using This service allowed multiple site like Way2sms, FullOnSMS, 160by2, Ultoo.com, SmsSpark.com SMSFI.com Freesms8, Site2SMS, IndyaRocks.com SMSAbc.com , Sms440, BhokaliSMS, etc.
In order to send sms you have to follow this three steps
1. Register at Site2SMS and get Username and Password.
2. Register at Mashape.com and generate key.
3. Download the .DLL file from ASPSnippets.SmsAPI.dll click here and add it in your project bin folder
error report in line no 27 : give solution
ReplyDeleteThe remote server returned an error: (403) Forbidden.
Line 25: {
Line 26: //Single SMS
Line 27: SMS.SendSms(txtRecieveNumber.Text.Trim(), txtMessage.Text.Trim());
Line 28: }
Line 29: else
I am not getting any error nor sending message.please help me.
ReplyDeleteplz read the step clearly and then try again it will work....thank you
Deletecheck your network connection and plz read the step clearly and then try again it will work....thank you
Deletei have follow all steps correctly and got mashap key but key doesn't work for me. {"message":"Invalid Mashape Key"} this error accure.
DeleteThis comment has been removed by the author.
ReplyDeleteCan I get rid of BLZ that comes in every SMS that I send through this API??
ReplyDeleteplz follow this step carefully
DeleteRegister at Site2SMS and get Username and Password.
2. Register at Mashape.com and generate key
thanks...you are doing a great job..keep it up..
ReplyDeleteThe remote server returned an error: (403) Forbidden.
ReplyDeleteiam getting this error on cs.aspx.cs line 23
it was working fine.....plz follow this step carefully
DeleteRegister at Site2SMS and get Username and Password.
2. Register at Mashape.com and generate key
and then try....
from where will i get the AspSnippets.SMsAPI class? plz help
ReplyDeleteit is .dll file you can download from this path
Deletehttp://aspsnippets.com/DownloadFile.aspx?File=ASPSnippets.SmsAPI.dll#center
thanks alot.
DeleteStill i get an error on thus line:
SMS.SendSms(txtRecipientNumber.Text.Trim(), txtMessage.Text.Trim());
An exception of type 'System.Net.WebException' occurred in System.dll but was not handled in user code
Additional information: The remote server returned an error: (403) Forbidden.
Plzz help
it will work fine so plz check your internet connection or follow the step properly that describes in this post and the try again it will work for me very nicely.....thank you
DeleteHow to get API key
DeleteRegister at http://docs.mashape.com/api-keys and generate key.
Deletehow to add dll file to bin folder we dont have any bin folder
ReplyDeleteplease reply to us early..........
Create bin Folder in your project and put this .dll file in this folder thank you........
Deletehii kirit sir...
ReplyDeleteI done all the process as u said...but WngMdwqbqDfZjhftJFb6FMKUAPqU this is my key...where should it paste...
error 403 getting....thank you
which key we have to copy testing key or production key...
ReplyDeletePaste ur testing key in place of maspee key in a code
ReplyDeletehii kirit sir...
ReplyDeleteI done all the process as u said...but WngMdwqbqDfZjhftJFb6FMKUAPqU this is my key...where should it paste...
error 403 getting....thank you
which key we have to copy testing key or production key...
after sending sms ..there is no error ...also msg not getting to receiver,,..after using break point ..
ReplyDeletetry
{
send("8421621475", "swapnil", txtMessageBody.Text,txtmobno.Text);
from here jump to catch...plz help
either sir give mail id I will sent all coding with snapshot...thank you
Hii ...
ReplyDeleteiam getting this error;
The remote server returned an error: (403) Forbidden.
try
{
SMS.APIType = SMSGateway.Site2SMS;
SMS.MashapeKey = "";
SMS.Username = "my number";//Site2SMS username
SMS.Password = "my passward"//;Site2SMS passward
SMS.SendSms("recivernumber", EmailBody);
}
catch (Exception ex)
{
//ex message =The remote server returned an error: (403) Forbidden.
}
And i m Register at Site2SMS also
Deleteand Register at Mashape.com
and get testing key
SMS.MashapeKey = "";
it will work properly follow the main three steps properly and the try again and also mobile can't have DND support oterwise message will not send.
Deletefinally done..thnx
DeleteThis comment has been removed by the author.
ReplyDeleteH! Kirit awesome blog ...your code is working 100% perfect ....
ReplyDeletethank you bro...... most welcome
Deletehello rahul sir, please help me why i got following error
Delete{"message":"Invalid Mashape Key"}
not working bro... i have to do with generated key?..
ReplyDeletefor bidden error(403).. what i have to do with generated key in mashape?..
ReplyDeleteit's working for all user plz follow the step properly and the try again thank you.......
Deleteit works , but how to send using ultoo.com
ReplyDeletePlease help.......................
it will only support this site only
DeleteWay2sms, FullOnSMS, 160by2, Ultoo.com, SmsSpark.com SMSFI.com Freesms8, Site2SMS, IndyaRocks.com SMSAbc.com , Sms440, BhokaliSMS, etc.
I just want send sms world wide not only in india
ReplyDeletethen purchase your own Gateway and sending sms anyway......thank you.....
DeleteThanks for ur code., It's working perfectly.,
ReplyDeletecan u tell me how to send SMS using postmethod? and how to save the nos separately into database?
ReplyDeletethnx bro its working ............... thnx.........
ReplyDeleteSMS.SendSms(txtRecieveNumber.Text.Trim(), txtMessage.Text.Trim());
ReplyDeleteThe remote certificate is invalid according to the validation procedure.
This is the error
how to get the connections.pls help me Mr.kirit kapupara
ReplyDeletePlease tell me the Reference Name
ReplyDeleteIts work very fine and what connection plz mentioned your comments briefly so I can understand.
DeleteSite2Sms website is not running on google...Blody site
ReplyDeleteerror - The underlying connection was closed: The connection was closed unexpectedly.
ReplyDeletecomplete all steps
rply: ketan_padhiyar@ymail.com
SMS.SendSms(txtRecieveNumber.Text.Trim(), txtMessage.Text.Trim());
ReplyDeletethis error has occured please tell me what to do..
please mail me anujkeshri10@gmail.com
The remote server returned an error: (403) Forbidden.
ReplyDeletehi i like it........
DeleteHi Sir,
ReplyDeleteAm getting error like this,
The remote server returned an error: (503) Server Unavailable.
Hi KIRIT,
ReplyDeletethanks for great article ...
Welcome and also view blog update post and support this blog .
DeleteThanks for the FANTASTIC post! This information is really good information about Bulk SMS ProvidersI m looking forward desperately for the next post of yours.
ReplyDeleteRegards,
Bulk SMS Hyderabad
hi.. can you please help with the vb .net code for this
ReplyDeleteSame code just you have to change your syntax from c# to VB else nothing other change.
ReplyDeleteThanks for your prompt reply.. will try to find the syntax changes..
ReplyDeleteCan you please provide the steps for generating the test key in mashape
ReplyDeleteLogin in mashpe and copy the test key and paste it in your code
Deletei have registered and created user name and password.. but i am not sure how to generate the key.. also do we need to register any api ? i am not clear on this part.. if you can help with the steps.. that will be of great help..
Deletethanks mate.. i was able to find it out..thanks so much for the post. I am able to successfully send the sms using vb application.
Deletehi kirit gr8 nice post!
ReplyDeletebut i have one requirement is there any chance to sending message with out registration ?????
because my requirement is we need to sending message from my database mobile numbers like who are users in my database
you have any idea for that one?
thank u
You can send SMS easily by just fetch mobile number of the user which are located in your database. Thank you plz put comment if you have any doubt and support the blog
DeleteThis comment has been removed by the author.
ReplyDeleteyou have to first register with this number that is in database.suppose you register with 9978718547 then also put the entry of this number in database and then now you can send sms to any other user by this number.
ReplyDeletenope i want to send by my database numbers
Deletesuppose i have one user record inmy db his number is somethink like 9999999999 if he is login to my application he is sending message by his number only it should by 9999999999
this is possible but before 9999999999 user login he is must register with Site2SMS and mashpee website.
ReplyDeleteyeah thats my question is there any chance to(user 9999999999) send sms with out registration is there any dlls for that one
DeleteSorry no idea about that thanks...
ReplyDeleteThis comment has been removed by the author.
DeleteNo idea about this website
ReplyDeleteI followd the same steps.I can able to send message both single person and group .
ReplyDeleteThanks a lot ..
Hi Thanks for this post. It is workable to me.
ReplyDeleteBulk SMS Hyderabad
Thank you and also touch with us for new post
Delete..
please tell me how can I get test keys in mashape.com.kindly help me
Deletehow can I get test keys in mashape.com and i have already registered and couldn't find that keys.
ReplyDeleteJust login in mashpee and copy test key and paste in your code.
Deleteyeah this really working.Actually I am developing a project.My project is about to send notification about any event which are added by the user through email and msg.can u pls tell me how to implement this in my project.
DeleteI am unable to send sms to any other number except mine..
ReplyDeleteKindly help
May be they have activated DND service.So send sms to those number without DND service.
DeleteHi I'm getting this error do u have any solution for this.....
ReplyDeleteThe remote server returned an error: (403) Forbidden.
It is work fine plz follow the proper step.
ReplyDeletewhen login in http://www.mashape.com/keys
ReplyDelete404 page not found display
Thank you very much. Your posting is very great.
ReplyDeleteBulk sms in Bhubaneswar
Bulk SMS Service Provides a unique opportunity to e-commerce website where they can bridge the gap for customer service by offering SMS Service Technology. It is very useful for us.Thanks for Sharing. Bulk Sms Services
ReplyDeleteThe information about Bulk SMS given in this article was awesome. Thanks for sharing with us.
ReplyDeletebulk sms services in Indore | bulk sms services provider
I am unable to generate key please help..
ReplyDeleteI am unable to generate key please help..
ReplyDeleteSimply follow the step as define in above description.
DeleteUseful information.
ReplyDeleteHello sir. i implemented all code. it's working fine for same state only
ReplyDeleteAs my account on site2sms is haryana mobile number. this code send sms to haryana numbers only not to other state numbers.
please help me with that
i want to send messages to other states also
DeleteYou have to pay for this services.
ReplyDeleteand i also want to ask that this .DLL file supports only site2sms. doesn't support other websites like way2sms, 160by2 is there any solution if i want to use some other website
DeleteNo other site not provide facility.
DeleteBulk SMS Marketing is one of the most practical options to market your product. Bulk SMS are best way to communicate personal computer to mobile phone and bulk smsbulk sms has become the most effective, cheap and reliable means of communication, advertising, marketing, campaigning also. Thanks for sharing...
ReplyDeleteBulk SMS Marketing Services across India
Thanks for comment.
DeleteI could not Getting this error
ReplyDeleteASP.NET runtime error: Could not load file or assembly 'ASPSnippets.SmsAPI (4)' or one of its dependencies. The located assembly's manifest definition does not match the assembly reference. (Exception from HRESULT: 0x80131040) D:\SQL Quries\windows connection\practice\SMS\SMS\WebForm1.aspx 1 1 SMS
sir i want to change header and remove the add from sms so how can we do that
ReplyDeleteThat is not possible because this is provided by SMS facility free so simply it will use its header in every SMS that you send.
ReplyDeleteawesome. it is working. it is perfect. thank you very much.
ReplyDeleteam getting this kind of error so please tell me the answer...................the error is
ReplyDeleteError 1 The type or namespace name 'ASPSnippets' could not be found (are you missing a using directive or an assembly reference?) C:\Users\ARUN\Desktop\dm1\Default3.aspx.cs 10 7 C:\...\dm1\
Add asp snippets DLL file in your bin folder.
ReplyDeleteI have done every thing carefully, yet it is showing an error "Invalid Mashape key"
ReplyDeletemy error .Mashape key is invaild
ReplyDeletehi friend i have some error in my proj
ReplyDeleteSMS.SendSms(txtRecieveNumber.Text.Trim(), txtMessage.Text.Trim());
ReplyDeletethis line error for me
SMS.SendSms(txtRecieveNumber.Text.Trim(), txtMessage.Text.Trim());
ReplyDeleteerror;
this api is deprecated due to take down request from site2sms
how to solve this problem
Hi generate proper key and follow the proper step.
Deletegeting following error This API is Deprecated due to take down request from site2sms can u help
Deletei got this error.........
ReplyDeleteplz give me solution sir
response":"This API is Deprecated due to take down request from site2sms
how to genrate proper key. lot off time we are genrate the key but not take proper solution..plz help me
ReplyDeleteget following error
ReplyDeleteThis API is Deprecated due to take down request from site2sms
getting following error
ReplyDeleteThis API is Deprecated due to take down request from site2sms
can u help
its showing ,message":"Invalid Mashape Key".. please help me ..
ReplyDeleteit showing = {"message":"Invalid Mashape Key"} error
ReplyDeleteit showing = {"message":"Invalid Mashape Key"} error
ReplyDeleteplease help
I Am Getting {"message":"Invalid Mashape Key"} ..
ReplyDeletePlz Help.....
Not working error is occur. error is invalid Mashape Key
ReplyDeleteI have all the steps i.e 1. Registered with way2sms and 2. Mashape.com
ReplyDeleteand created Mashape Key there are two types of keys Testing & Production
Tried both but getting same error below with both keys
System.Exception: {"message":"Invalid Mashape Key"}
Any Idea?
Hi
ReplyDeleteKirit Sir,
i am gettng error invalid mashupkey ..i am using above code and testing mashupkey..then what's the problem ?
http://www.designsmatrix.in
Hello Sir,
ReplyDeleteI am getting Invalid Mashape Key Error when sending sms.I am not understanding what to do.Please help.
You can immediately access the service http://www.intistele.com/ and fast connect API SMS in your personal account. This is the perfect solution for you if you don't want to mess with code.
ReplyDeletethanks for inform. also visit blog regularly for new update.
Deleteit's perfect share about "Bulk SMS" ,..
ReplyDeleteThanks a lot for sharing this perfect article about "Bulk SMS" ,
ReplyDeletehello,mine says invalid mashape key can u tell me how to get this key.....i logged in and created a application and clicked get the keys...
ReplyDeletehow do i get joomla bulk sms component?
ReplyDeletebulk sms service
thanks and also share blog with your students for new updates.
ReplyDeletethanks for comments.
ReplyDeletehow i get Mashape Key
ReplyDeletewhere i get Mashape Key
can u please provide the Mashape Key
hi register in mashpee website and create application and get the api or mashpee key.yhank you.
DeleteI followed all steps and getting runtime error "message":"Invalid Mashape Key" .Please suggest ..
ReplyDeleteNice Article..and Doing Bulk SMS Business is Very Effective..Todays advertising Field If we Talk about fatest and high conversion medium of marketing than it is Bulk SMS Marketing..if you are looking Bulk SMS company in Jaipur than Please Visit us..
ReplyDeleteAadishesh - the finest bulk SMS service provider in India! Use our SMS gateways to send bulk SMS Online anywhere in India at economical prices!
ReplyDeleteTake advantage of a free test account before acquiring your package. Only finest routes used for national, international and DND numbers. Special plans for promotional & transactional bulk SMS. Order your pack today and redeem countless benefits right away! Aadishesh Info Communications
SMSGATEWAYHUB offers users to send SMS from Excel sheet directly. As most Business Professionals use MS Excel for organizing their customer data; SMSc, along with its feature to send SMS from Excel, empowers all users to save their precious time and send personalized bulk SMS.
ReplyDeleteBulk SMS Services From Pnpuniverse provides web using http and smpp server shift through the DND data and TRAI rules by sending sms to simply choice base.
ReplyDeleteBulk SMS Service
i got the below error :
ReplyDelete{"message":"Invalid Mashape Key"}
same error here bro,.
Deletewhat's solution for this
same error how to slove this
Deletei got the below error :
ReplyDelete{"message":"Invalid Mashape Key"}
any solutions for that
mr.kirit kapupara
please reply fast
Deletem also getting the same error {"message":"Invalid Mashape Key"}
ReplyDeleteI followed the entire procedure...help
Same error [Exception: {"message":"Invalid Mashape Key"}]
ReplyDeleteis this API still valid. or just a junk of code.
i have followed all the step and also generate the key but i got error msg {"message":"Invalid Mashape Key"}
ReplyDeletei am also getting the same error
ReplyDelete{"message":"Invalid Mashape Key"}
I have the same error
ReplyDelete{"message":"Invalid Mashape Key"}
any one solved this error ?
I have the same error
ReplyDelete{"message":"Invalid Mashape Key"}
pls solve the error sir
I have the same error..
Delete{"message":"Invalid Mashape Key"}
I also have the same error..
Delete{"message":"Invalid Mashape Key"}
i genrate mashape key but {"message":"Invalid Mashape Key" error
ReplyDeletei will try all the way to sort out this error... everything is well but message cant sent to any number... There is no error seen but cant send messages.... What i do now///
ReplyDeleteIn This World of Communication and fast moving business through web and internet it becomes very essential to save the time for marketing and promotion of your products and business. So, there is an easy solution for all the entrepreneurs to promote their new products over the market with the help of Bulk SMS Technique.
ReplyDeletei genrate mashape key but {"message":"Invalid Mashape Key" error
ReplyDeleteThanks a lot for sharing such a good source with all, i appreciate your efforts taken for the same. I found this worth sharing and must share this with all.can u visit us
ReplyDeleteivr services in india
Thanks for sharing great thought, I like your blog post very much.
ReplyDeleteSend Bulk Business SMS Indore
this is only for india ....how can i use this for Pakistan......
ReplyDeletegive me possible solution
sorry I have no idea about it.
DeleteHi sir , am getting the error {"message":"Invalid Mashape Key"} even i generated Mashape Key. Please help me as soon as possible.
ReplyDeleteThanks for providing great information. I like your post very much.
ReplyDeleteSMS Scheduling
Very Nice Information. Thanks for share
ReplyDeletePhD Thesis Writing Services in India
Dissertation Writing Services in India
Research Paper Writing Services in India
M.tech Thesis Writing Services in India
This comment has been removed by the author.
ReplyDeletenice post you post is very useful and interesting
ReplyDeleteGet My Ex Back
Get My Wife Back
Get My Husband Back
I Want My Wife Back
I Want My Husband Back
Love Problem Specialist
Have My Love Stop Being Angry On Me
Love Marriage Solution Specialist
How To Convince Boyfriend/Girlfriend To Get Marry
How To Make My Husband Listen To Me
Extra Affair And Relationship Solution By Vashikaran
Stop Separtion And Divorce Problem
How Can I Impress My Girlfreind
How Can Make My Wife In Our Favour
How Can I Attract My Ex
How Can Stop Marriage Of My Lover
Get Some One Back Spells
followed all the steps. not getting any error but the message is not delivered.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteIt is always difficult to send bulk messages to a large number of people but this article has provided a long lasting solution to this problem using the #C and VB.Net. We have employed the bulk SMS service to send message to our clients who are accessing our Letter of Recommendation Writing Services
ReplyDeleteNot working
ReplyDeletesame bro pls reply
ReplyDeleteI have the same error..
ReplyDelete{"message":"Invalid Mashape Key"}
Approve Adsense accounts
ReplyDeletenice post
ReplyDeleteSms Gateway Api
thanks. also keep visiting updated post on blog.
DeleteIf you are a software developer and you need to be used to the common programming practices, you should be used to using such forums. ASP.NET is one of the programming languages which are in high demand. I have passion and with time I will like to use this programming language. Could you be writing a programming journal? Ask for professional Journal Article Critiquing Help.
ReplyDeleteThanks for writing this in-depth post. You covered every angle. The great thing is you can reference different parts.
ReplyDeleteBest Bulk SMS in Hyderabad
hi ,i got the error"{"message":"Invalid Mashape Key"}",
ReplyDeleteplease suggest??
Using bulk SMS softwares is always a good idea to quickly initiate the Bulk SMS sending. These softwares mostly resides in the operators network and manages the processes.
ReplyDeleteSEO Company In Indore
Bulk SMS Provider is a premium SMS gateway. Our services can be used for SMS marketing Chennai. Services include bulk SMS, voice SMS, reseller and API added. Bulk SMS service providers in Chennai.
ReplyDeleteThanks for sharing the blog regarding the Bulk SMS service provider. Keep sharing more and more.
ReplyDeleteBulk SMS is utilized as promotional SMS and Transactional for SMS marketing. Our bulk SMS marketing solution helps you to send cheap bulk promotional SMS service provider in India. It's least expensive marketing solution to reach achieve imminent clients. MAXWELL Communication furnishes bulk SMS service with NDNC filter, so you don't have any lawful issues with NDNC Registry.Best Bulk Sms Provider In India
ReplyDeleteThanks for sharing valuable information. OneXtel is the best Bulk SMS Provider and across India providing bulk sms services at best prices with reliable Gateways and APIs.
ReplyDeleteBulk SMS Providers Hyderabad gives you the access to establish communication, market your goals and gain more consumers, bulk sms 1India's number bulk SMS Hyderabad.
ReplyDeleteBulk SMS Providers Hyderabad
Search your mobile number is registered or not
ReplyDeletePlease check the following link
DND Check
Very nice article about software and related information, its very nice article. thanks for sharing such great article hope kee sharing such kind of article Android bulk sms sender
ReplyDeleteI really like your post..Bulk SMS becoming important services for business promotion.. it is really very effective for companies to grow faster..thanks for sharing such a great post!!
ReplyDeleteNice Sharing,Bulk SMS is the right way to reach your target customer, but to choice right provider is important and if you wish to try ‘’MsgClub’’ services then there is no other platform better than this.Bulk SMS API
ReplyDeleteWay2SMS is well known bulk sms service provider and thanks for update.Bulk SMS API
ReplyDeleteIts very nice informative article. thanks for sharing such great article hope keep sharing such kind of article Best android bulk sms sender software
ReplyDeleteThanks for sharing such great article hope keep sharing such kind of article Bulk Sms Sender Software
ReplyDeletebulk sms services
ReplyDeletebulk sms provider in india
Tree SMS is the best Bulk SMS Service provider company in India Our followng services are-
ReplyDeleteBulk SMS service provider in India
Bulk SMS services in India
Transactional Bulk SMS service in India
Promotional SMS service in India
Bulk SMS Service in Uttarakhand
Bulk SMS Service Provider in Dehradun
bulk SMS services in Uttar Pradesh
Bulk SMS services in Delhi NCR
Hey, very nice site. I came across this on Google, and I am stoked that I did. I will definitely be coming back here more often. Wish I could add to the conversation and bring a bit more to the table, but am just taking in as much info as I can at the moment. Thanks for sharing.
ReplyDeleteDai Software