Description:-
In this
example we explain that how to perform Asynchronous delete operation using Ajax
in MVC4.
With the AJAX approach, the user clicks a “delete” link and
is then presented with a JavaScript confirmation. If the user confirms,
an asynchronous call is sent to a controller action to handle the delete.
When the controller action completes, a callback is made to a JavaScript
function which updates the UI by removing the deleted row from the HTML
table. It sounds pretty fancy but MVC makes it quite simple. I
should be clear, what I’m doing here isn’t clever and certainly is not
unique. I originally got the idea for this off some other blog a few
years back. I’d love to give that person credit but I’ve long since lost
the link. Mostly I’m just posting this here as a reference for myself
since it seems my need to do this sort of thing comes up infrequently enough
that whenever I do need to do it, I’ve since forgotten how.
In the project I created for this post, I’m using the MVCContrib grid, just for ease of use when it comes to laying out a model in a table. I’m also using Twitter Bootstrap, mostly because it’s my most recent obsession and I really like how easy it is to use it to style a web app. Bootstrap is doing nothing more than styling the table, it’s not performing any functional role in this example. The code for the view ends up looking like this:
Check File type before upload Upload only word and image file in asp.net
how to upload image in database have a datatype is Image upload image with image datatype
How to create or generate Captcha code in asp.net captcha code same like Google in asp.net
to download complete example click below download image link
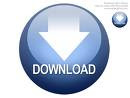
Homecontroller.cs:-
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Net;
using
System.Web;
using
System.Web.Mvc;
using
ThirteenDaysAWeek.AjaxDelete.Web.Models;
namespace
ThirteenDaysAWeek.AjaxDelete.Web.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
ViewBag.Message = "Modify this template
to jump-start your ASP.NET MVC application.";
IList<Product> model = new List<Product>
{
new Product
{
ProductId = 1,
ProductName = "Big Widget",
UnitPrice = 2.99m
},
new Product
{
ProductId = 2,
ProductName = "Medium Widget",
UnitPrice = 1.99m
},
new Product
{
ProductId = 3,
ProductName = "Small Widget",
UnitPrice = .99m
}
};
return View(model);
}
[AcceptVerbs(HttpVerbs.Delete)]
public ActionResult Delete(int id)
{
// TODO:
Add some implementation here that deletes the specified object
// from your repository.
// Return the id of the object we
deleted. This gets passed into our
callback javascript function
// so we know what table row to remove
if (id == 2)
{
return new HttpStatusCodeResult(HttpStatusCode.InternalServerError, "Something went wrong");
}
else
{
return Json(new { id = id });
}
}
public ActionResult About()
{
ViewBag.Message = "Your app description
page.";
return View();
}
public ActionResult Contact()
{
ViewBag.Message = "Your contact
page.";
return View();
}
}
}
Index.cshtml:-
@using MvcContrib.UI.Grid
@model IList<ThirteenDaysAWeek.AjaxDelete.Web.Models.Product>
@{
ViewBag.Title = "Home Page";
}
<link rel="stylesheet" href="@Url.Content("~/Content/bootstrap.css")"/>
<script type="text/javascript" src="@Url.Content("~/Scripts/jquery-1.7.1.js")"></script>
<script type="text/javascript" src="@Url.Content("~/Scripts/jquery.unobtrusive-ajax.js")"></script>
<script type="text/javascript" src="@Url.Content("~/Scripts/bootstrap.js")"></script>
@Html.Grid(Model).Columns(cb
=>
{
cb.For(product
=> @Ajax.ActionLink("Delete", "Delete", new {id = product.ProductId},
new AjaxOptions
{
Confirm = "Are you sure you want
to delete this product?",
HttpMethod = "DELETE",
OnSuccess
= "onProductDeleteSuccess",
OnFailure = "onProductDeleteFailure"
}));
cb.For(product
=> product.ProductName)
.Named("Product Name");
cb.For(product
=> product.UnitPrice)
.Named("Unit Price");
}).Attributes(new Dictionary<string, object>
{
{"class", "table table-striped"}
}).RowAttributes(data
=> new Dictionary<string, object>
{
{"id", string.Concat("product-id-", data.Item.ProductId)}
})
<script type="text/javascript">
// Callback function that is invoked when
our Delete operation succeeds
function onProductDeleteSuccess(response, status, data) {
var id = "#product-id-" + response.id;
$(id).remove();
}
function onProductDeleteFailure(context) {
alert("Something went horribly wrong");
}
</script>
thank you Bro.......
ReplyDelete