Description:-
In this example we explain that how to create controls dynamically like TextBox,DropdownList etc… in asp.net and retrieve the values of the dynamically created control and access it.
In which example we created Textbox for name field,another Textbox for salary field and DropdownList for age field and when user enter data into this dynamic control and click on submit button the data is inserted in database and bind it in Gridview Dynamically.
Look at this user enter 5 in textbox then 5 rows dynamically created and fillup the data and click on submit button then all 5 rows will be inserted in database.
Ajax password checker example Check the Strength of Password using ajax in asp.net
How to handle concurrency in Linq to sql Concurrency Example linq to sql in asp.net
Example of Royal flush in css Royal flush example using CSS
to download complete Example click below download link
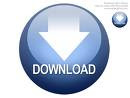
Default3.aspx:-
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default3.aspx.cs" Inherits="Default3" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox><asp:Button ID="Button2" runat="server" Text="Button" OnClick="Button2_Click" />
<asp:Table id="Table1" runat="server" BorderColor="Black" BorderStyle="Solid" BorderWidth="3px" Font-Bold="True" >
</asp:Table>
<asp:PlaceHolder id="PlaceHolder1" runat="server"></asp:PlaceHolder><BR><BR>
<br />
<asp:GridView ID="GridView1" runat="server"></asp:GridView>
</form>
</body></html>
Default3.aspx.cs:-
using
System;
using
System.Web.UI.WebControls;
using
System.Data;
using
System.Data.SqlClient;
public partial class Default3 :
System.Web.UI.Page
{
protected void Page_Load(object
sender, EventArgs e)
{
if (ViewState["Count"] != null)
{
AddTextBoxs();
AddButton();
}
}
private void AddTextBoxs()
{
clear();
int cnt = Convert.ToInt32(TextBox1.Text);
TableHeaderRow htr = new TableHeaderRow();
TableHeaderCell htc1 = new TableHeaderCell();
htc1.Width =
100;
TableHeaderCell htc2 = new TableHeaderCell();
htc2.Width =
100;
TableHeaderCell htc3 = new TableHeaderCell();
htc3.Width =
50;
htc1.Text = "Name";
htc2.Text = "Salary";
htc3.Text = "Year";
htr.Cells.Add(htc1);
htr.Cells.Add(htc2);
htr.Cells.Add(htc3);
Table1.Rows.Add(htr);
for (int i = 0; i < cnt; i++)
{
TableRow tr = new TableRow();
TableCell tc1 = new TableCell();
tc1.Width = 100;
TextBox t = new TextBox();
t.Width
= 100;
t.ID = "txtnm" + Table1.Rows.Count;
tc1.Controls.Add(t);
TableCell tc2 = new TableCell();
TextBox t1 = new TextBox();
t1.Width
= 100;
tc2.Width = 100;
t1.ID = "txtsal" + Table1.Rows.Count;
tc2.Controls.Add(t1);
TableCell tc3 = new TableCell();
DropDownList dpl = new DropDownList();
dpl.Width = 50;
tc3.Width = 50;
dpl.ID =
"dpl" + Table1.Rows.Count;
for (int j = 0; j < 10; j++)
dpl.Items.Add(j.ToString());
tc3.Controls.Add(dpl);
tr.Cells.Add(tc1);
tr.Cells.Add(tc2);
tr.Cells.Add(tc3);
Table1.Rows.Add(tr);
}
}
private void AddButton()
{
Button b = new Button();
b.ID = "btn";
b.Text = "Button";
b.Click += new System.EventHandler(btn_Click);
PlaceHolder1.Controls.Add(b);
}
protected void Button1_Click(object sender, EventArgs e)
{
AddTextBoxs();
if (ViewState["Count"] == null) AddButton();
ViewState["Count"] = Convert.ToInt16(ViewState["Count"]) + 1;
}
protected void btn_Click(object
sender, EventArgs e)
{
SqlConnection cn = new SqlConnection("Data Source=SQLDB;User
ID=Demoh;Password=Demo1@");
cn.Open();
for (int i = 1; i < Table1.Rows.Count; i++)
{
//string dp =
((TextBox)Table1.Rows[i+1].FindControl("txtnm" + i+1)).Text;
TextBox tnm = (TextBox)Table1.Rows[i].FindControl("txtnm" + i);
TextBox tsal = (TextBox)Table1.Rows[i].FindControl("txtsal" + i);
DropDownList tyear = (DropDownList)Table1.Rows[i].FindControl("dpl" + i);
SqlCommand cmd = new SqlCommand("insert into poll values('" + tnm.Text + "','" + tsal.Text + "','" + tyear.SelectedValue + "')", cn);
cmd.ExecuteNonQuery();
bindgrid();
}
clear();
}
protected void Button2_Click(object sender, EventArgs e)
{
// AddButton();
clear();
AddTextBoxs();
if (ViewState["Count"] == null) AddButton();
ViewState["Count"] = Convert.ToInt16(ViewState["Count"]) + 1;
}
public void bindgrid()
{
SqlConnection cn = new SqlConnection("Data Source=SQLDB;User
ID=Demoh;Password=Demo1@");
SqlCommand cmd = new SqlCommand("select * from poll", cn);
DataTable dt = new DataTable();
SqlDataAdapter sa = new SqlDataAdapter();
cn.Open();
sa.SelectCommand = cmd;
cmd.ExecuteNonQuery();
sa.Fill(dt);
GridView1.DataSource = dt;
GridView1.DataBind();
}
public void clear()
{
Table1.Rows.Clear();
for (int i = 0; i < Table1.Rows.Count; i++)
Table1.Rows.RemoveAt(i);
}
}
Hello Everyone..U should try this also...
ReplyDeleteI have created one post for the same that generate the controls dynamically and also saves the values from that. If you have no problem then follow this link: http://goo.gl/U6Zfk4