Description:-
Here I will explain how to use Json and Jquery to submit the Form
without page refresh using Ajax inasp.net
In previous articles I explained How to Call Server Side Metod or code Behind Method using Json and Jquery in Asp.Net . Now I will explain how to submit the Form without refresh or reload page and also Insert,Update,Delete in Gridview without Refresh the Page using Json/Jquery in asp.net.
To implement
this Program first you have to Create a
table with name SampleInfoTable in your database like below to save
values in database.
In this Example
we provide Facility to user to perform a CRUD operation in Gridview and
maintain data in Sqlserver Database without Refresh the page is simply by using
the Functionality of Ajax and Json/Jquery.
To show Example How to insert,update,delete in DataList control clikck here CRUD operation in DataList
How to Bind Excelsheet Data to Gridview CRUD operation in Excelsheet Database
How to Display Layer on one Another in CSS Overlapping Layer in CSS
To show Example How to insert,update,delete in DataList control clikck here CRUD operation in DataList
How to Bind Excelsheet Data to Gridview CRUD operation in Excelsheet Database
How to Display Layer on one Another in CSS Overlapping Layer in CSS
to Download Complete example click the Below download Image link
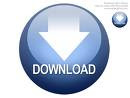
Default.aspx:-
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0
Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.tableStyle
{
background-color: White;
border: 1px solid rgb(204, 204, 204); font-family: Tahoma;
font-size: 14px; width: 1000px; border-collapse: collapse;
}
.tableHeader
{color: White; height:30px; background-color: rgb(15, 159, 15); font-weight: bold;
}
.tableRaw
{border: 1px solid rgb(204, 204, 204);
}
input.button
{
font: bold 12px Arial, Sans-serif;
height: 24px;
margin: 0;
padding: 2px 3px;
color: black;
border: none;
}
</style>
<script src="scripts/jquery-1.4.3.min.js" type="text/javascript"></script>
<script type="text/javascript">
$(document).ready(function () {
// Load Information from database at the
page loading
GetEmployeeDetails();
});
//
// This is select method for JSON
//
function GetEmployeeDetails() {
$.ajax({
type: "POST",
url:
"hr_webservice.asmx/GetEmployeeDetails",
data: "{}",
contentType: "application/json;
charset=utf-8",
dataType: "json",
success: function (response) {
BindTable(response.d);
},
failure: function (msg) {
alert(msg);
}
});
}
//
// Validation check before insert
//
function BlankValidation(editedName, editedEmail, editedPhone,
editedAddress) {
var returnVal = true;
if (editedName.value == '') {
alert('Blank is not allow');
editedName.focus();
returnVal = false;
return returnVal;
}
else if (editedEmail.value == '') {
alert('Blank is not allow');
editedEmail.focus();
returnVal = false;
return returnVal;
}
else if (editedPhone.value == '') {
alert('Blank is not allow');
editedPhone.focus();
returnVal = false;
return returnVal;
}
else if (editedAddress.value == '') {
alert('Blank is not allow');
editedAddress.focus();
returnVal = false;
return returnVal;
}
return returnVal;
}
//
// Edit Employee Data
//
function EditEmployeeData() {
var editedName = document.getElementById('txEditedName');
var editedEmail = document.getElementById('txEditedEmail');
var editedPhone = document.getElementById('txEditedPhone');
var editedAddress =document.getElementById('txEditedAddress');
var hfEditedId = document.getElementById('hfEditedId');
var hfActionMode = document.getElementById('hfActionMode');
// Blank validation check
if (BlankValidation(editedName, editedEmail, editedPhone, editedAddress)
== true) {
// call ajax JSON method
$.ajax({
type: "POST",
url:
"hr_webservice.asmx/EditEmployeeData",
data: "{ 'employeeId':
'" + hfEditedId.value
+ "','editedName':
'" + editedName.value
+ "', 'editedEmail':
'" + editedEmail.value
+ "','editedPhone':'" + editedPhone.value
+ "','editedAddress':'" + editedAddress.value
+ "','action_mode':'" + hfActionMode.value + "'}",
contentType: "application/json;
charset=utf-8",
dataType: "json",
success: function (response) {
BindTable(response.d);
},
failure: function (msg) {
alert(msg);
}
});
ResetText();
}
}
//
// Reset after action
//
function ResetText() {
var editedName = document.getElementById('txEditedName');
var editedEmail = document.getElementById('txEditedEmail');
var editedPhone = document.getElementById('txEditedPhone');
var editedAddress = document.getElementById('txEditedAddress');
var hfEditedId = document.getElementById('hfEditedId');
var hfActionMode = document.getElementById('hfActionMode');
var btnEntry = document.getElementById('btnEntry');
btnEntry.value = "New Entry";
hfActionMode.value = "NEW";
editedName.value = '';
editedEmail.value
= '';
editedPhone.value = '';
editedAddress.value = '';
hfEditedId.value = '0';
}
//
// While the Edit button clicks, the
values sets on entry fields, at the same time the button
// name also changed to UPDATE and Hidden field value also changed to
UPDATE. When I will sent the hidden field
// to procedure as action mode, the
procedure will work for update
//
function EditMode(empId, empName, empEmail, empPhone, empAddress)
{
var hfEditedId = document.getElementById('hfEditedId');
var txEditedName = document.getElementById('txEditedName');
var txEditedEmail = document.getElementById('txEditedEmail');
var txEditedPhone = document.getElementById('txEditedPhone');
var txEditedAddress = document.getElementById('txEditedAddress');
var hfActionMode = document.getElementById('hfActionMode');
var btnEntry = document.getElementById('btnEntry');
hfEditedId.value = empId;
txEditedName.value = empName;
txEditedEmail.value = empEmail;
txEditedPhone.value = empPhone;
txEditedAddress.value = empAddress;
hfActionMode.value = "UPDATE";
btnEntry.value = "Update";
}
//
// This is delete mode with JSON, When
the button fire the onclick event the
// method call with the hidden primary
key.
// actually the hidden field set on
create the row.
//
function DeleteMode(deleteEmpId) {
var agree = confirm("Are you sure you want
to delete this information ?");
if (agree) {
$.ajax({
type:
"POST",
url: "hr_webservice.asmx/DeleteEmployeeData",
data: "{ 'employeeId':
'" + deleteEmpId + "'}",
contentType: "application/json;
charset=utf-8",
dataType: "json",
success: function (response) {
BindTable(response.d);
},
failure: function (msg) {
alert(msg);
}
});
}
}
//
// The method used to generate the grid
with table, This method fire when the
// JSON find the well serealized data
from webService.
//
function BindTable(Employees) {
var root = document.getElementById('mydiv');
try {
var tblId = document.getElementById('tblGridValue');
if (tblId != null) {
root.removeChild(tblId);
}
}
catch (e)
{
}
var tab = document.createElement('table');
tab.setAttribute("id", "tblGridValue");
tab.setAttribute("class", "tableStyle");
tab.setAttribute("cellspacing", "3px");
var tbo = document.createElement('tbody');
var row, cell;
// the list object now extract the value
for each row
$.each(Employees, function (index, employee) {
row
= document.createElement('tr');
row.setAttribute("class", "tableRaw");
//
// the object of LIST is now extract the
each cell of row
//
for (var j = 0; j < 5; j++) {
cell = document.createElement('td');
cell.setAttribute("width", "200px");
var empId = employee.id;
var empName = employee.Name;
var empEmail = employee.Email;
var empPhone = employee.Phone;
var empAddress = employee.Address;
if (j == 0) {
//Create an input type
dynamically.
var hiddenId = document.createElement("input");
//Assign different attributes to the element.
hiddenId.setAttribute("type", "hidden");
hiddenId.setAttribute("id", "hfRow_" + employee.Id);
hiddenId.setAttribute("value", employee.Id);
cell.appendChild(hiddenId);
cell.appendChild(document.createTextNode(employee.Name));
}
else if (j == 1) {
var spanValue = document.createElement("span");
cell.setAttribute("width", "200px");
spanValue.setAttribute("display", "inline-block");
spanValue.appendChild(document.createTextNode(employee.Email));
cell.appendChild(spanValue);
}
else if (j == 2) {
cell.setAttribute("width", "200px");
cell.appendChild(document.createTextNode(employee.Phone));
}
else if (j == 3) {
cell.setAttribute("width", "200px");
cell.appendChild(document.createTextNode(employee.Address));
}
else if (j == 4) {
//
// in this state loop
generates Edit and Delete button for each row
//
var element = document.createElement("img");
element.setAttribute("src", "images/edit-icon.gif");
element.setAttribute("width", "15px");
cell.setAttribute("width", "100px");
//
// This loop also adding a
click event EditMode()
//
element.setAttribute("onclick", "EditMode('"
+ empId + "','"
+ empName + "','"
+ empEmail + "','"
+ empPhone + "','"
+
empAddress + "')");
cell.appendChild(element);
//
// Same way the row created
Delete button
//
var elementDelete = document.createElement("img");
elementDelete.setAttribute("src", "images/DeleteRed.png");
elementDelete.setAttribute("width", "15px");
//
// Also created the Delete
Method in onclick event
//
elementDelete.setAttribute("onclick", "return
DeleteMode('" + empId + "')");
cell.appendChild(elementDelete);
}
row.appendChild(cell);
}
tbo.appendChild(row);
});
tab.appendChild(tbo);
root.appendChild(tab);
}
</script>
</head>
<body style="background-color:#cccccc;">
<form id="form1" runat="server" >
<div style="float:left; background-color:White; width:1050px;">
<br />
<br />
<div style="background-color:White">
<div style="clear:both"></div>
</div>
<div style="width:1000px; background-color:White;">
<table class="tableStyle" width="1000px" >
<tbody>
<tr style="border:1px solid black;">
<td style="width:200px">
<asp:HiddenField id="hfEditedId" runat="server" />
<asp:HiddenField id="hfActionMode" Value="NEW" runat="server" />
<input id="txEditedName" type="text" name="name" value=" " style="width:98%" />
</td>
<td style="width:200px">
<input id="txEditedEmail" type="text" name="name" value=" " style="width:98%" />
</td>
<td style="width:200px">
<input id="txEditedPhone" type="text" name="name" value=" " style="width:98%" />
</td>
<td style="width:200px">
<input id="txEditedAddress" type="text" name="name" value=" " style="width:98%" />
</td>
<td style="width:100px; padding-left:10px;">
<div style="padding-bottom:5px">
<input id="btnEntry" type="button" class="button" name="editAjaxGrid" value="New Entry" style="width:80px" onclick="EditEmployeeData()" />
</div>
<div style="padding-bottom:5px">
<input id="Button1" type="button" class="button" name="reset" value="Reset" style="width:80px" onclick="
ResetText()" />
</div>
</td>
</tr>
<tr class="tableHeader">
<td style="width:200px">
Name
</td>
<td style="width:200px">
Email
Address
</td>
<td style="width:200px">
Mobile Number
</td>
<td style="width:200px">
Address
</td>
<td style="width:100px; padding-left:10px;">
Action</td>
</tr>
</tbody>
</table>
<div id="mydiv">
</div>
</div>
</div>
</form>
</body>
</html>
hr_webservice.asmx:-
<%@ WebService Language="C#" CodeBehind="hr_webservice.asmx.cs" Class="hr_webservice" %>
hr_webservice.asmx.cs:-
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Web.Services;
using
System.Data;
using
System.Data.SqlClient;
using
System.Web.Configuration;
/// <summary>
/// Summary description for
EmployeeWebService
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To allow this Web Service to be called from script,
using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class hr_webservice : System.Web.Services.WebService
{
List<Employee> list = new List<Employee>();
public hr_webservice()
{
}
//
// Get Employee List from Database, This
is a sample code for getting the data from database, you can use procedure to
get insted of
// inline query
//
[WebMethod]
public List<Employee>
GetEmployeeDetails()
{
// getting connection string
string conStr = WebConfigurationManager.ConnectionStrings["TESTDB"].ConnectionString;
DataTable dt = new DataTable();
SqlDataReader dr = null;
// Creating Sql Connection
using (SqlConnection conn = new SqlConnection(conStr))
{
// Creating insert statement
string sql = string.Format(@"Select * from [SampleInfoTable]");
SqlCommand cmd = new SqlCommand();
cmd.Connection = conn;
cmd.CommandText = sql;
cmd.CommandType = CommandType.Text;
conn.Open();
dr =
cmd.ExecuteReader();
dt.Load(dr);
conn.Close();
cmd = null;
}
int countRow = dt.Rows.Count;
foreach (DataRow drEmp in dt.Rows)
{
Employee objemployee = new Employee();
objemployee.id = Convert.ToInt32(drEmp["id"].ToString());
objemployee.Name = drEmp["Name"].ToString();
objemployee.Email = drEmp["Email"].ToString();
objemployee.Phone = drEmp["Phone"].ToString();
objemployee.Address = drEmp["Address"].ToString();
list.Add(objemployee);
}
return list;
}
[WebMethod]
public List<Employee>
DeleteEmployeeData(string employeeId)
{
// getting connection string
string conStr = WebConfigurationManager.ConnectionStrings["TESTDB"].ConnectionString;
int rowsInserted = 0;
// Creating Sql Connection
using (SqlConnection conn = new SqlConnection(conStr))
{
// Creating insert statement
string sql = string.Format(@"Delete [SampleInfoTable] WHERE id='" + employeeId + "'");
SqlCommand
cmd = new SqlCommand();
cmd.Connection = conn;
cmd.CommandText = sql;
cmd.CommandType = CommandType.Text;
conn.Open();
rowsInserted = cmd.ExecuteNonQuery();
conn.Close();
cmd = null;
}
list =
GetEmployeeDetails();
return list;
}
//
// Edit Employee Data
//
[WebMethod]
public List<Employee>
EditEmployeeData(string employeeId, string editedName, string editedEmail, string editedPhone, string editedAddress, string action_mode)
{
// getting connection string
string conStr = WebConfigurationManager.ConnectionStrings["TESTDB"].ConnectionString;
int rowsInserted = 0;
// Creating Sql Connection
using (SqlConnection conn = new SqlConnection(conStr))
{
string query = "insert into
SampleInfoTable values('" +
editedName + "','" + editedEmail + "','" + editedPhone + "','" + editedAddress + "')";
SqlCommand cmd = new SqlCommand(query,conn);
conn.Open();
rowsInserted = cmd.ExecuteNonQuery();
conn.Close();
cmd = null;
}
list = GetEmployeeDetails();
return list;
}
}
public class Employee
{
public int id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string Phone { get; set; }
public string Address { get; set; }
}
0 comments:
Post a Comment