Description:-
In
this Example I will Expalin that How to Upload Word File,ExcelFile or Any other
type of File in sql server Database and again Download the same File From
SqlServer Database.
in which we will Bind the All information of
uploaded file in Gridview when user upload the file. The information of File
means Type,Name,Size,Desc etc.
when user upload the File then this Record are
Automatically Bind to the Gridview with a Download Link.so user Click on the
Download Button then this File are Downloded.
To implement this Program First you have to create a
Table in Database
Table
Name:- dbtodownload
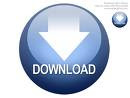
To Show Example of How to Upload File in MVC Application then click here upload Image and bind to Gridview in MVC
To show Example of How to send SMS in Asp.net click here Send SMS to user mobile in Application
How to upload File and Fetch file from SqlServer and bind to Gridview fetch file from Sqlserver and bind to Gridview
uploadfileindbanddown.aspx:-
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="uploadfileindband
down.aspx.cs" Inherits="uploadfileindband_down" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:FileUpload ID="fileUpload1" runat="server" /><br />
<asp:Button ID="btnUpload" runat="server" Text="Upload" onclick="btnUpload_Click" />
</div>
<div>
<asp:GridView ID="Griddata" runat="server" AutoGenerateColumns="false" DataKeyNames="Id">
<HeaderStyle BackColor="#df5015" Font-Bold="true" ForeColor="White" />
<Columns>
<asp:BoundField DataField="Id" HeaderText="Id" />
<asp:BoundField DataField="FileName" HeaderText="FileName" />
<asp:BoundField DataField="FileType" HeaderText="FileType" />
<asp:BoundField DataField="FileDesc" HeaderText="FileDescription" />
<asp:TemplateField HeaderText="FilePath">
<ItemTemplate>
<asp:LinkButton ID="lnkDownload" runat="server" Text="Download" OnClick="lnkDownload_Click"></asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</div>
</form>
</body>
</html>
uploadfileindbanddown.aspx.cs:-
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Web.UI;
using
System.Web.UI.WebControls;
using
System.Data;
using
System.Data.SqlClient;
using
System.IO;
public partial class uploadfileindband_down :
System.Web.UI.Page
{
string
strCon = "Data Source=SQLDB;Initial
Catalog=Demo;User ID=Demoh;Password=Demo1@";
protected void
Page_Load(object sender, EventArgs e)
{
if
(!IsPostBack)
{
BindGrid();
}
}
// Bind Gridview Data
private void
BindGrid()
{
using (SqlConnection
con = new SqlConnection(strCon))
{
using (SqlCommand
cmd = new SqlCommand())
{
cmd.CommandText = "select
* from dbtodownload";
cmd.Connection = con;
con.Open();
Griddata.DataSource = cmd.ExecuteReader();
Griddata.DataBind();
con.Close();
}
}
}
// Save files to Folder and
files path in database
protected void
btnUpload_Click(object sender, EventArgs e)
{
string
filename = Path.GetFileName(fileUpload1.PostedFile.FileName);
Stream
str = fileUpload1.PostedFile.InputStream;
BinaryReader br
= new BinaryReader(str);
Byte[]
size = br.ReadBytes((int)str.Length);
using (SqlConnection
con = new SqlConnection(strCon))
{
using (SqlCommand
cmd = new SqlCommand())
{
cmd.CommandText = "insert
into dbtodownload(FileName,FileType,FileData,FileDesc)
values(@Name,@Type,@Data,@Desc)";
cmd.Parameters.AddWithValue("@Name",
filename);
cmd.Parameters.AddWithValue("@Type", "application/word");
cmd.Parameters.AddWithValue("@Data",
size);
cmd.Parameters.AddWithValue("@Desc", "hi
this is a file");
cmd.Connection = con;
con.Open();
cmd.ExecuteNonQuery();
con.Close();
BindGrid();
}
}
}
// This button click event is
used to download files from gridview
protected void
lnkDownload_Click(object sender, EventArgs e)
{
LinkButton
lnkbtn = sender as LinkButton;
GridViewRow
gvrow = lnkbtn.NamingContainer as GridViewRow;
int
fileid = Convert.ToInt32(Griddata.DataKeys[gvrow.RowIndex].Value.ToString());
string
name, type;
using (SqlConnection
con = new SqlConnection(strCon))
{
using (SqlCommand
cmd = new SqlCommand())
{
cmd.CommandText = "select
FileName, FileType, FileData,FileDesc from dbtodownload where Id=@Id";
cmd.Parameters.AddWithValue("@id",
fileid);
cmd.Connection = con;
con.Open();
SqlDataReader dr
= cmd.ExecuteReader();
if
(dr.Read())
{
Response.ContentType = dr["FileType"].ToString();
Response.AddHeader("Content-Disposition", "attachment;filename=\"" +
dr["FileName"] + "\"");
Response.BinaryWrite((byte[])dr["FileData"]);
Response.End();
}
}
}
}
}
0 comments:
Post a Comment