Description:-
In
this Example we Explain that How to Create Registration Form with Client and Server
side Validation in MVC4.Generally in which we include all control validation
like Email validation,phone number validation,password validation,DropdownList
validation and CheckBox validation.
We all know that Registration form is a common form and used
in Every Application , so that reason I want to demonstrate the basic validation in mvc4
webform.
There are number of step to devlop the Registration form
with Validation are as follows:
First in Home controller write the code for Bind the DropdownList of the Country and state just copy the below code and paste it.
Example for Insert,Update,Delete without Refreshing the webpage CRUD operation without refreshing the page using Json jquery
How to Bind Dynamic data in Ajax Accordion Control Bind data from database in Accordion control
to Download Complete Example click below Download link
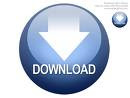
HomeController:-
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using Mvc4_RegistrationForm.Models;
using System.Text.RegularExpressions;
namespace Mvc4_RegistrationForm.Controllers
{
public class HomeController : Controller
{
#region
Private Methods
void BindCountry()
{
List<Country> lstCountry = new List<Country>{new Country { ID = null, Name = "Select" },
new Country { ID = 1, Name = "India" },
new Country { ID = 2, Name = "USA" }
};
ViewBag.Country = lstCountry;
}
//for
server side
void BindCity(int? mCountry)
{
try
{
if (mCountry != 0)
{
//below code is only for demo, since city list will come
from database based on country
//Hence remove below lines of code when you will query
data from city table with in database based on country
int index = 1;
List<City> lstCity = new List<City>{
new City
{
Country = 0,
ID=null, // using
index++ for making unique ID for city
Name = "Select"
},
new City
{
Country = 1,
ID=index++, // using
index++ for making unique ID for city
Name = "Delhi"
},
new City
{
Country = 1,
ID=index++,
Name = "Lucknow"
},
new City
{
Country = 1,
ID=index++,
Name = "Noida"
},
new City
{
Country = 1,
ID=index++,
Name = "Guragon"
},
new City
{
Country = 1,
ID=index++,
Name = "Pune"
},
new City
{
Country = 2,
ID=index++,
Name = "New-York"
},
new City
{
Country = 2,
ID=index++,
Name = "California"
},
new City
{
Country = 2,
ID=index++,
Name = "Washington"
},
new City
{
Country = 2,
ID=index++,
Name = "Vermont"
},
};
var city = from c in lstCity
where c.Country ==
mCountry || c.Country == 0
select c;
ViewBag.City = city;
}
else
{
List<City> City = new List<City> { new City { ID = null, Name = "Select" } };
ViewBag.City = City;
}
}
catch (Exception ex)
{
}
}
#endregion
//for
client side using jquery
public JsonResult CityList(int mCountry)
{
try
{
if (mCountry != 0)
{
//below code is only for demo, since city list will come
from database based on country
//Hence remove below lines of code when you will query
data from city table with in database based on country
int index = 1;
List<City> lstCity = new List<City>{
new City
{
Country = 0,
ID=null, // using
index++ for making unique ID for city
Name = "Select"
},
new City
{
Country = 1,
ID=index++, // using
index++ for making unique ID for city
Name = "Delhi"
},
new City
{
Country = 1,
ID=index++,
Name = "Lucknow"
},
new City
{
Country = 1,
ID=index++,
Name = "Noida"
},
new City
{
Country = 1,
ID=index++,
Name = "Guragon"
},
new City
{
Country = 1,
ID=index++,
Name = "Pune"
},
new City
{
Country = 2,
ID=index++,
Name = "New-York"
},
new City
{
Country = 2,
ID=index++,
Name = "California"
},
new City
{
Country = 2,
ID=index++,
Name = "Washington"
},
new City
{
Country = 2,
ID=index++,
Name = "Vermont"
},
};
var city = from c in lstCity
where c.Country ==
mCountry || c.Country == 0
select c;
// return
Json(city, JsonRequestBehavior.AllowGet);
return Json(new SelectList(city.ToArray(), "ID", "Name"), JsonRequestBehavior.AllowGet);
}
}
catch (Exception ex)
{
}
return Json(null);
}
public ActionResult Index()
{
return View();
}
public ActionResult Demo()
{
Response.Redirect("http://www.dotnet-tricks.com/Tutorial/mvclist");
return View();
}
public ActionResult RegistrationForm()
{
BindCountry();
BindCity(0);
return View();
}
[HttpPost]
public ActionResult RegistrationForm(RegistrationModel mRegister)
{
//Check
Server Side Validation by using data annotation
if (ModelState.IsValid)
{
return View("Completed");
}
else
{
//
To DO: if client validation failed
ViewBag.Selpwd =
mRegister.Password; //for setting password value
ViewBag.Selconfirmpwd =
mRegister.ConfirmPassword;
ViewBag.SelCountry =
mRegister.Country; // for setting selected
country
BindCountry();
ViewBag.SelCity =
mRegister.City;// for setting selected city
if (mRegister.Country != null)
BindCity(mRegister.Country.ID);
else
BindCity(null);
return View();
}
}
}
}
now create the RegistrationModel class in Model Folder and paste the below code.
RegistrationModel:-
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.ComponentModel.DataAnnotations;
using
System.Web.Mvc;
using
System.Text.RegularExpressions;
namespace
Mvc4_RegistrationForm.Models
{
public class RegistrationModel
{
[Required(ErrorMessage = "Please Enter Email Address")]
[Display(Name = "UserName")]
[RegularExpression(".+@.+\\..+", ErrorMessage = "Please Enter Correct
Email Address")]
public string UserName { get; set; }
[Required(ErrorMessage = "Please Enter Password")]
[StringLength(50, ErrorMessage = "The {0} must be at least {2}
characters long.", MinimumLength = 6)]
[DataType(DataType.Password)]
[Display(Name = "Password")]
public string Password { get; set; }
[Required(ErrorMessage = "Please Enter Confirm Password")]
[StringLength(50, ErrorMessage = "The {0} must be at least {2}
characters long.", MinimumLength = 6)]
[DataType(DataType.Password)]
[Display(Name = "Confirm
password")]
[Compare("Password", ErrorMessage = "The password and confirmation
password do not match.")]
public string ConfirmPassword { get; set; }
[Display(Name = "Country")]
public Country Country { get; set; }
[Display(Name = "City")]
public City City { get; set; }
[Required(ErrorMessage = "Please Enter Address")]
[Display(Name = "Address")]
[StringLength(200)]
public string Address { get; set; }
[Required(ErrorMessage
= "Please Enter Mobile
No")]
[Display(Name = "Mobile")]
[StringLength(10, ErrorMessage = "The Mobile must contains 10
characters", MinimumLength = 10)]
public string MobileNo { get; set; }
[MustBeTrue(ErrorMessage = "Please Accept the Terms & Conditions")]
public bool TermsAccepted { get; set; }
}
public class MustBeTrueAttribute : ValidationAttribute, IClientValidatable // IClientValidatable for
client side Validation
{
public override bool IsValid(object value)
{
return value is bool && (bool)value;
}
// Implement IClientValidatable for
client side Validation
public IEnumerable<ModelClientValidationRule> GetClientValidationRules(ModelMetadata metadata, ControllerContext context)
{
return new ModelClientValidationRule[] { new ModelClientValidationRule { ValidationType = "checkbox", ErrorMessage = this.ErrorMessage } };
}
}
public class MustBeSelected : ValidationAttribute, IClientValidatable // IClientValidatable for
client side Validation
{
public override bool IsValid(object value)
{
if (value == null || (int)value == 0)
return false;
else
return true;
}
// Implement IClientValidatable for
client side Validation
public IEnumerable<ModelClientValidationRule> GetClientValidationRules(ModelMetadata metadata, ControllerContext context)
{
return new ModelClientValidationRule[] { new ModelClientValidationRule { ValidationType = "dropdown", ErrorMessage = this.ErrorMessage } };
}
}
public class Country
{
[MustBeSelected(ErrorMessage = "Please Select Country")]
public int? ID { get; set; }
public string Name { get; set; }
}
public class City
{
[MustBeSelected(ErrorMessage = "Please Select City")]
public int? ID { get; set; }
public string Name { get; set; }
public int? Country { get; set; }
}
}
now create the view or RegistrationForm.cshtml Under Home Folder in your Application and paste the below code
RegistrationForm.cshtml:-
@model Mvc4_RegistrationForm.Models.RegistrationModel
@{
ViewBag.Title = "Registration Form";
}
<script src="../../Scripts/jquery-1.7.1.min.js" type="text/javascript"></script>
<script src="../../Scripts/jquery.validate.min.js" type="text/javascript"></script>
<script src="../../Scripts/jquery.validate.unobtrusive.min.js" type="text/javascript"></script>
<script type="text/jscript">
jQuery.validator.unobtrusive.adapters.add("dropdown", function (options) {
//
debugger;
if (options.element.tagName.toUpperCase() == "SELECT" &&
options.element.type.toUpperCase() == "SELECT-ONE") {
options.rules["required"] = true;
if (options.message) {
options.messages["required"] = options.message;
}
}
});
jQuery.validator.unobtrusive.adapters.add("checkbox", function (options) {
if (options.element.tagName.toUpperCase() == "INPUT" && options.element.type.toUpperCase()
== "CHECKBOX") {
options.rules["required"] = true;
if (options.message) {
options.messages["required"] = options.message;
}
}
});
$(function () {
$('#Country_ID').change(function ()
{
//
debugger;
var id = $("#Country_ID
:selected").val();
if (id != "") {
$.ajax({
type: "GET",
contentType: "application/json;
charset=utf-8",
url: '@Url.Action("CityList", "Home")',
data: { "mCountry": id },
dataType: "json",
beforeSend: function () {
//alert(id);
},
success: function (data) {
var items = "";
$.each(data, function (i, city) {
items += "<option
value='" + city.Value + "'>" + city.Text + "</option>";
});
$('#City_ID').html(items);
},
error: function (result) {
alert('Service call failed: ' + result.status + ' Type :' + result.statusText);
}
});
}
else {
var items = '<option
value="">Select</option>';
$('#City_ID').html(items);
}
});
});
</script>
<h2>
Registration
Form with Client & Server Side Validation</h2>
@using (Html.BeginForm())
{
<fieldset>
<legend></legend>
<ol>
<li>
@Html.LabelFor(m => m.UserName)
@Html.TextBoxFor(m => m.UserName, new { maxlength = 50 })
@Html.ValidationMessageFor(m => m.UserName)
</li>
<li>
@Html.LabelFor(m => m.Password)
@Html.PasswordFor(m => m.Password, new { maxlength = 50, value = ViewBag.Selpwd
})
@Html.ValidationMessageFor(m => m.Password)
</li>
<li>
@Html.LabelFor(m => m.ConfirmPassword)
@Html.PasswordFor(m => m.ConfirmPassword, new { maxlength = 50, value = ViewBag.Selconfirmpwd })
@Html.ValidationMessageFor(m => m.ConfirmPassword)
</li>
<li>
@Html.LabelFor(m => m.Country)
@Html.DropDownListFor(m => m.Country.ID, new SelectList(ViewBag.Country, "ID", "Name", ViewBag.SelCountry), new { style = "width:310px" })
@Html.ValidationMessageFor(m => m.Country.ID)
</li>
<li>
@Html.LabelFor(m => m.City)
@Html.DropDownListFor(m => m.City.ID, new SelectList(ViewBag.City, "ID", "Name", ViewBag.SelCity), new { style = "width:310px" })
@Html.ValidationMessageFor(m => m.City.ID)
</li>
<li>
@Html.LabelFor(m => m.Address)
@Html.TextAreaFor(m => m.Address, new { maxlength =200 })
@Html.ValidationMessageFor(m => m.Address)
</li>
<li>
@Html.LabelFor(m => m.MobileNo)
@Html.TextBoxFor(m =>
m.MobileNo ,new { maxlength = 10 })
@Html.ValidationMessageFor(m => m.MobileNo)
</li>
<li>
@Html.CheckBoxFor(m => m.TermsAccepted) I accept the terms &
conditions
@Html.ValidationMessageFor(m
=> m.TermsAccepted)
</li>
</ol>
<input type="submit" value="Submit" />
</fieldset>
}
thanx
ReplyDeletehelp for your blogger.
you welcome Bro......
DeleteHow we can use @HTML.validationMessage instead of @HTML.ValidationMessageFor.
ReplyDelete