Description:-
In this
Example we explain that How to Create Crud(create,Read,update,Delete) operation
in Modal Popup in Mvc4 Application. Previous time we Explain a lot of example
of Mvc now we Create a DML operation in Gridview using Modal popup in MVC
Application.
First you have to create a Table name like customer
as following structure:
Here is Some Image or Scrren shot of this Application at runtime are as follows:-
1. Validation Screen Shot:-
Create Operation,Update,Delete operation:-
In this Example we use Jquery For Creating a Insert
upadate Delete Operation in Modal Popup.and we Create a Three Modal popup for
Create and Edit,Upade, Delete. When user Create an Create link the new Popup
windows is Appear with Creating a Form Design of Craete record same Process for
the Edit Update and Delete Operation.
Implement Remember Me functionality using CheckBox ASP.Net
set WaterMark Text in PDF using itextsharp in C#
How to set Default Button in MVC Web Form Application
How to Bind XML File data to Treeview in asp.net
JQuery datepicker calender with Dropdown month and year in asp.net.
To Download The Complete Project Click the below Download Image Link
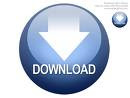
Step 1:- First you to Add Linq to Sql class means Dbml file and Add the customer table in it.
Step 2:- Add the customerModel class in Model Folder or Direcory and write the Following Code.
customerModel.cs:-
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations;
using System.Web.Mvc;
using System.Text.RegularExpressions;
using Mvc4_CRUD_jQueryDialog.Models;
//Required for custom paging
using System.Linq.Expressions;
namespace Mvc4_CRUD_jQueryDialog.Models
{
public class ModelServices : IDisposable
{
private readonly DataEntities entities = new DataEntities();
DataClasses1DataContext dc = new DataClasses1DataContext();
public IEnumerable<customer> GetCustomer()
{
return dc.customers.ToList();
// return entities.Customers.ToList();
}
//For Custom Paging
public IEnumerable<customer> GetCustomerPage(int pageNumber, int pageSize, string searchCriteria)
{
if (pageNumber < 1)
pageNumber = 1;
return dc.customers
// .OrderBy(searchCriteria)
.Skip((pageNumber - 1) *
pageSize)
.Take(pageSize)
.ToList();
}
public int CountCustomer()
{
return dc.customers.Count();
}
public void Dispose()
{
entities.Dispose();
}
//For Edit
public customer GetCustomer(int mCustID)
{
return dc.customers.Where(m => m.c_id ==
mCustID).FirstOrDefault();
}
public bool CreateCustomer(customer mCustomer)
{
try
{
dc.customers.InsertOnSubmit(mCustomer);
dc.SubmitChanges();
return true;
}
catch (Exception mex)
{
return false;
}
}
public bool UpdateCustomer(customer mCustomer)
{
try
{
customer data = dc.customers.Where(m => m.c_id
== mCustomer.c_id).FirstOrDefault();
data.c_address =
mCustomer.c_address;
data.c_mobile =
mCustomer.c_mobile;
data.c_name = mCustomer.c_name;
dc.SubmitChanges();
return true;
}
catch (Exception mex)
{
return false;
}
}
public bool DeleteCustomer(int mCustID)
{
try
{
customer data = dc.customers.Where(m => m.c_id
== mCustID).FirstOrDefault();
dc.customers.DeleteOnSubmit(data);
dc.SubmitChanges();
return true;
}
catch (Exception mex)
{
return false;
}
}
}
//public enum SortDirection
//{
// Ascending,
// Descencing
//}
public class PagedCustomerModel
{
public int TotalRows { get; set; }
public IEnumerable<customer> Customer { get; set; }
public int PageSize { get; set; }
}
public class customerModel
{
[ScaffoldColumn(false)]
public int c_id { get; set; }
[Required(ErrorMessage = "Please Enter Name")]
public string c_name { get; set; }
[Required(ErrorMessage = "Please Enter Address")]
[UIHint("Multiline")]
public string c_address { get; set; }
[Required(ErrorMessage = "Please Enter Contact No")]
public string c_mobile { get; set; }
}
}
HomeController:-
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using Mvc4_CRUD_jQueryDialog.Models;
namespace Mvc4_CRUD_jQueryDialog.Controllers
{
public class HomeController : Controller
{
ModelServices mobjModel = new ModelServices();
public ActionResult Index()
{
return View();
}
public ActionResult Demo()
{
Response.Redirect("http://www.dotnet-tricks.com/Tutorial/mvclist");
return View();
}
//Bind Web Grid and also do paging
public ActionResult WebGrid(int page = 1, string sort = "c_name", string sortDir = "ASC")
{
const int pageSize = 5;
var totalRows = mobjModel.CountCustomer();
sortDir = sortDir.Equals("desc", StringComparison.CurrentCultureIgnoreCase)
? sortDir : "asc";
var validColumns = new[] { "c_id", "c_name", "c_address", "c_mobile" };
if (!validColumns.Any(c =>
c.Equals(sort, StringComparison.CurrentCultureIgnoreCase)))
sort = "custid";
var customer =
mobjModel.GetCustomerPage(page, pageSize, "it." + sort + " " + sortDir);
var data = new PagedCustomerModel()
{
TotalRows = totalRows,
PageSize = pageSize,
Customer = customer
};
return View(data);
}
public ActionResult Create()
{
if (Request.IsAjaxRequest())
{
customerModel cust = new customerModel();
ViewBag.IsUpdate = false;
return View("Add_Customer", cust);
}
else
return View();
}
public ActionResult View(int id)
{
var data = mobjModel.GetCustomer(id);
if (Request.IsAjaxRequest())
{
customerModel cust = new customerModel();
cust.c_id = data.c_id;
cust.c_name = data.c_name;
cust.c_address =
data.c_address;
cust.c_mobile = data.c_mobile;
return View("_ViewCustomer", cust);
}
else
return View(data);
}
public ActionResult Edit(int id)
{
var data = mobjModel.GetCustomer(id);
if (Request.IsAjaxRequest())
{
customerModel cust = new customerModel();
cust.c_id = data.c_id;
cust.c_name = data.c_name;
cust.c_address =
data.c_address;
cust.c_mobile = data.c_mobile;
ViewBag.IsUpdate = true;
return View("_Customer", cust);
}
else
return View(data);
}
public ActionResult Delete(int id)
{
bool check = mobjModel.DeleteCustomer(id);
var data = mobjModel.GetCustomer();
return RedirectToAction("WebGrid");
}
[HttpPost]
public ActionResult CreateEditCustomer(customerModel mCust, string Command)
{
// Validate the model being submitted
if (!ModelState.IsValid)
{
return PartialView("_Customer", mCust);
}
else if (Command == "Save")
{
customer mobjcust = new customer();
mobjcust.c_id = mCust.c_id;
mobjcust.c_address =
mCust.c_address;
mobjcust.c_mobile =
mCust.c_mobile;
mobjcust.c_name = mCust.c_name;
bool check =
mobjModel.CreateCustomer(mobjcust);
if (check)
{
TempData["Msg"] = "Data has
been saved succeessfully";
ModelState.Clear();
return RedirectToAction("WebGrid", "Home");
}
}
else if (Command == "Update")
{
customer mobjcust = new customer();
mobjcust.c_id = mCust.c_id;
mobjcust.c_address =
mCust.c_address;
mobjcust.c_mobile =
mCust.c_mobile;
mobjcust.c_name = mCust.c_name;
bool check =
mobjModel.UpdateCustomer(mobjcust);
if (check)
{
TempData["Msg"] = "Data has
been updated succeessfully";
ModelState.Clear();
return RedirectToAction("WebGrid", "Home");
}
}
return PartialView("_Customer");
}
}
}
WebGrid.cshtml:-
@model Mvc4_CRUD_jQueryDialog.Models.PagedCustomerModel
@{
ViewBag.Title = "Web Grid";
WebGrid grid = new WebGrid(rowsPerPage: Model.PageSize);
grid.Bind(Model.Customer,
autoSortAndPage: false,
rowCount: Model.TotalRows
);
}
<link href="@Url.Content("~/Content/themes/base/minified/jquery-ui.min.css")" rel="stylesheet" type="text/css" />
<script src="@Url.Content("~/Scripts/jquery-1.7.1.min.js")" type="text/javascript"></script>
<script src="@Url.Content("~/Scripts/jquery-ui-1.8.20.min.js")" type="text/javascript"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#CreateCustomer").live("click", function (e) {
// e.preventDefault(); use this or return
false
var url = $(this).attr('href');
$("#dialog-edit").dialog({
title: 'Create Customer',
autoOpen: false,
resizable: false,
height: 355,
width: 400,
show: { effect: 'drop', direction: "up" },
modal: true,
draggable: true,
open: function (event, ui) {
$(this).load(url);
},
close: function (event, ui) {
$(this).dialog('close');
}
});
$("#dialog-edit").dialog('open');
return false;
});
$(".editDialog").live("click", function (e) {
// e.preventDefault(); use this or return
false
var url = $(this).attr('href');
$("#dialog-edit").dialog({
title: 'Edit Customer',
autoOpen: false,
resizable: false,
height: 355,
width: 400,
show: { effect: 'drop', direction: "up" },
modal: true,
draggable: true,
open: function (event, ui) {
$(this).load(url);
},
close: function (event, ui) {
$(this).dialog('close');
}
});
$("#dialog-edit").dialog('open');
return false;
});
$(".confirmDialog").live("click", function (e) {
// e.preventDefault(); use this or return
false
var url = $(this).attr('href');
$("#dialog-confirm").dialog({
autoOpen: false,
resizable: false,
height: 170,
width: 350,
show: { effect: 'drop', direction: "up" },
modal: true,
draggable: true,
buttons: {
"OK": function () {
$(this).dialog("close");
window.location = url;
},
"Cancel": function () {
$(this).dialog("close");
}
}
});
$("#dialog-confirm").dialog('open');
return false;
});
$(".viewDialog").live("click", function (e) {
// e.preventDefault(); use this or return
false
var url = $(this).attr('href');
$("#dialog-view").dialog({
title: 'View Customer',
autoOpen: false,
resizable: false,
height: 250,
width: 400,
show: { effect: 'drop', direction: "up" },
modal: true,
draggable: true,
open: function (event, ui) {
$(this).load(url);
},
buttons: {
"Close": function () {
$(this).dialog("close");
}
},
close: function (event, ui) {
$(this).dialog('close');
}
});
$("#dialog-view").dialog('open');
return false;
});
$("#btncancel").live("click", function (e) {
// location.reload(true);
$("#dialog-edit").dialog('close');
});
});
</script>
<h2>
CRUD Operations using jQuery dialog and WebGrid</h2>
<br />
<div style="color: Green; font-weight: bold">
@TempData["msg"]
</div>
<br />
@grid.GetHtml(
fillEmptyRows: false,
alternatingRowStyle: "alternate-row",
headerStyle: "grid-header",
footerStyle: "grid-footer",
mode: WebGridPagerModes.All,
firstText: "<<
First",
previousText: "<
Prev",
nextText: "Next
>",
lastText: "Last
>>",
columns: new[] {
grid.Column("c_id",
header: "c_id", canSort: false),
grid.Column("c_name",
header: "c_name",
format: @<text>
@Html.ActionLink((string)item.c_name, "View", new { id = item.c_id },
new { @class = "viewDialog" })</text>
),
grid.Column("c_address"),
grid.Column("c_mobile",
header: "c_mobile"
),
grid.Column("",
header: "Actions",
format: @<text>
@Html.ActionLink("Edit", "Edit", new { id = item.c_id },
new { @class = "editDialog"/*, data_dialog_id =
"edit-Dialog"*/ })
|
@Html.ActionLink("Delete", "Delete", new { id = item.c_id },
new { @class = "confirmDialog"})
</text>
)
})
<br />
<a id="CreateCustomer" href="..\Home\Create">Create Customer</a>
<div id="dialog-confirm" style="display: none">
<p>
<span class="ui-icon ui-icon-alert" style="float: left; margin: 0 7px 20px 0;"></span>
Are you sure to delete ?
</p>
</div>
<div id="dialog-edit" style="display: none">
</div>
<div id="dialog-view" style="display: none">
</div>
@*<div id="dialog-add"
style="display: none">
</div>*@
Index.cshtml:-
@{
ViewBag.Title = "Dot Net Tricks - MVC4 CRUD Operations Demo";
}
@section featured {
<section class="featured">
<div class="content-wrapper">
<hgroup class="title">
<h1>@ViewBag.Title</h1>
</hgroup>
<p>
To learn more about ASP.NET
MVC4 visit
<a href="http://aspsolutionkirit.blogspot.in" title="ASP.NET MVC4
Tutorial">All Example of Asp.Net and MVC</a>.
</p>
</div>
</section>
}
<div style="height: 320px;">
</div>
@model Mvc4_CRUD_jQueryDialog.Models.customerModel
@{
Layout = null;
}
<script src="@Url.Content("~/Scripts/jquery.validate.min.js")" type="text/javascript"></script>
<script src="@Url.Content("~/Scripts/jquery.validate.unobtrusive.min.js")" type="text/javascript"></script>
<script src="@Url.Content("~/Scripts/jquery.unobtrusive-ajax.min.js")" type="text/javascript"></script>
@*@using
(Ajax.BeginForm("EditCustomer", "Home", new AjaxOptions {
HttpMethod = "POST", UpdateTargetId = "edit-Dialog" }))*@
@using (Html.BeginForm("CreateEditCustomer", "Home", "POST"))
{
<fieldset>
<legend>Person</legend>
@* <div style="color: Green; font-weight:
bold">
@ViewBag.Msg
</div>*@
@if (ViewBag.IsUpdate == true)
{
@Html.HiddenFor(model => model.c_id)
}
<div class="editor-label">
@Html.LabelFor(model
=> model.c_name)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.c_name)
@Html.ValidationMessageFor(model =>
model.c_name)
</div>
<div class="editor-label">
@Html.LabelFor(model =>
model.c_address)
</div>
<div class="editor-field">
@Html.EditorFor(model =>
model.c_address)
@Html.ValidationMessageFor(model =>
model.c_address)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.c_mobile)
</div>
<div class="editor-field">
@Html.EditorFor(model =>
model.c_mobile)
@Html.ValidationMessageFor(model =>
model.c_mobile)
</div>
<br />
<div style="float: right">
@if (ViewBag.IsUpdate == true)
{
<button type="submit" id="btnUpdate" name="Command" value="Update" class="ui-button
ui-widget ui-state-default ui-corner-all ui-button-text-only">
<span class="ui-button-text">Update</span></button>
}
else
{
<button type="submit" id="btnSave" name="Command" value="Save" class="ui-button
ui-widget ui-state-default ui-corner-all ui-button-text-only">
<span class="ui-button-text">Save</span></button>
}
<button type="button" id="btncancel" class="ui-button
ui-widget ui-state-default ui-corner-all ui-button-text-only">
<span class="ui-button-text">Cancel</span></button>
</div>
</fieldset>
}
_ViewCustomer.cshtml:
@model Mvc4_CRUD_jQueryDialog.Models.customerModel
@{
Layout = null;
}
@using (@Html.BeginForm())
{
<fieldset>
<legend>Customer</legend>
<div class="editor-label">
@Html.LabelFor(model => model.c_name)
</div>
<div class="editor-field">
@Html.DisplayTextFor(model => model.c_name)
</div>
<div class="editor-label">
@Html.LabelFor(model =>
model.c_address)
</div>
<div class="editor-field">
@Html.DisplayTextFor(model =>
model.c_address)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.c_mobile)
</div>
<div class="editor-field">
@Html.DisplayTextFor(model =>
model.c_mobile)
</div>
</fieldset>
}
_Message.cshtml:-
@{
Layout = null;
}
<p>Your data has been updated</p>
<p><a href="javascript:void(0);" class="close">Close
Window</a></p>
I have search a long time like this article.
ReplyDeleteYou are the best!!!
most welcome.............
DeleteUnable to run ur source code in visual studio 2012 and i have SQL Server 2012
ReplyDeleteGreat article. I have an issue when click the X close of the modal the dialog script stop working. and I and redirect to the link page.
ReplyDeleteGreat MVC Tutorial..
ReplyDeleteFor More Web Apps visit http://www.ati-erp.com
Great Article Sir.. But finding some issue like we dnt hv to create view for Edit n New Customer please help for this issue..
ReplyDeleteThanks in advance...
Thank you . just put function same like insert dialog box.
ReplyDeleteSir thank Is this reply for me... i mean to my this question (But finding some issue like we dnt hv to create view for Edit n New Customer please help for this issue..
ReplyDelete)
Hi, Friend me the source code does not work in modal validation, I closed the window and opens to add, I can explain the lines for validation, thanks
ReplyDeletethat is worked for me very nice.... may be you have some mistake in code....
ReplyDeletetokat
ReplyDeleteankara
trabzon
istanbul
izmir
İTANJZ
Dereköy
ReplyDeleteÇamkule
Elmalı
Asartepe
Davutlar
VU4LA0
EskiÅŸehir
ReplyDeleteDenizli
Malatya
Diyarbakır
Kocaeli
5460K
Ankara
ReplyDeleteBolu
Sakarya
Mersin
Malatya
2X04RU
Diyarbakır
ReplyDeleteSamsun
Antep
Kırşehir
Konya
XİG
elazığ
ReplyDeletebitlis
mardin
kastamonu
van
BSHXF
7788C
ReplyDeleteBolu Parça Eşya Taşıma
Bilecik Lojistik
Adana Lojistik
Kayseri Evden Eve Nakliyat
Manisa Parça Eşya Taşıma
Tekirdağ Boya Ustası
MuÅŸ Lojistik
Referans KimliÄŸi Nedir
Yozgat Lojistik
06200
ReplyDeleteAntalya Şehir İçi Nakliyat
Kırşehir Şehirler Arası Nakliyat
Edirne Şehirler Arası Nakliyat
Giresun Evden Eve Nakliyat
Çerkezköy Marangoz
Kocaeli Evden Eve Nakliyat
Bingöl Evden Eve Nakliyat
Kütahya Şehir İçi Nakliyat
Sui Coin Hangi Borsada
2B47D
ReplyDeletebinance referans kodu
CBC16
ReplyDeletereferans kodu binance
783B9
ReplyDeletekırklareli görüntülü sohbet uygulama
parasız görüntülü sohbet
ardahan görüntülü sohbet kızlarla
Samsun Görüntülü Canlı Sohbet
erzincan canlı sohbet odaları
sesli sohbet odası
Istanbul Telefonda Kadınlarla Sohbet
Urfa Sohbet Muhabbet
görüntülü sohbet
2DDB0
ReplyDeleteSinop Bedava Sohbet
bingöl bedava görüntülü sohbet
adana ücretsiz sohbet sitesi
Osmaniye Canlı Görüntülü Sohbet Uygulamaları
mardin bedava sohbet siteleri
kırıkkale telefonda kızlarla sohbet
Kocaeli En İyi Ücretsiz Görüntülü Sohbet Siteleri
van en iyi görüntülü sohbet uygulaması
bilecik kadınlarla rastgele sohbet
38A9D
ReplyDeleteInstagram Takipçi Hilesi
Kripto Para Nasıl Üretilir
Kripto Para Kazanma
Bitcoin MadenciliÄŸi Siteleri
Youtube BeÄŸeni Hilesi
Binance Referans Kodu
Bitcoin Kazanma
Binance Borsası Güvenilir mi
Coin Kazanma
3FA6E
ReplyDeleteledger desktop
safepal
onekey
satoshi
galagames
arbitrum
aave
trezor suite
shiba
FBCAC
ReplyDeleteellipal
shiba
arbitrum
dappradar
metamask
uniswap
solflare
chainlist
layerzero